How to create Map Visualisation using D3.js
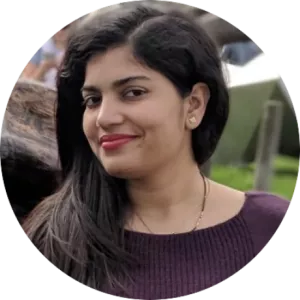
Nikita Sharma
Data Science InternD3.js is a javascript library used to create interactive data visualizations in web browsers. D3 is very powerful and can create numerous different visualizations. D3 has a lot of available charts that we can choose from for our visualizations.
In this post, we will be creating a basic map visualization using D3.js.
Getting started with D3js
Here we will see how to start with basic text in D3js. D3js is a javascript library that can be used inside Html for visualization. Here is a minimal HTML page with the D3 library imported.
Creating map visualization
So we know how to write simple text on D3js, now we will see how to create simple map visualization.
Create required styles
Here we will use CSS (Cascading Style Sheets) that is used to define the styles of the web-page.
In the above code, we have defined different styles of HTML elements. We can set different color, style, position, size, and many more to each of the element.
Create a canvas for our visualization
This is where we are going to create our map visualization.
Draw Australia Map boundaries
Here we are creating Australia Map boundaries by using D3 projection to locate Australia in the world map. Path generator will be used to locate the states of Australia which we will come soon. We are using a color range to fill different colors in different states. For now, we are just using 1 color but we can use a multi-color for every state.
Adding states to map with Geographic data
We need either geological or topological information to be able to plot the various states on the map. Thankfully this information is easily available in JSON format.
Here is a sample of Australia's geographical data, you can get the data from here.
We will load Geojson data in the following way.
Here we are creating the states in the map by loading the state location information. We are also extracting the state names from the loaded data and mentioning the names in the map.
All the code
So this is how we can create a basic Australia map using D3. We can also plot maps for other countries in the same way as Australia map with their geological data.
Finishing Note
In this post, we learned how to plot basic map visualization in D3js. In the next post we will see how we can plot points on the map and also how we can make our map interactive.